Front-end developer interview questions grouped into basic, intermediate, and advanced levels:
Basic:
1. What is HTML and what does it stand for?
2. What is CSS and what is its role in web development?
3. What is the difference between inline, block, and inline-block elements in CSS?
4. What is the box model in CSS?
5. What is the purpose of JavaScript in web development?
6. What are the different data types in JavaScript?
7. What is the difference between == and === in JavaScript?
8. What is a CSS preprocessor, and why would you use one?
9. What is responsive web design?
10. What is the purpose of media queries in CSS?
Intermediate:
1. What are the differences between GET and POST requests?
2. Explain the concept of closures in JavaScript.
3. What is event delegation in JavaScript?
4. How do you optimize website performance?
5. What are some common CSS frameworks and libraries, and when would you use them?
6. Explain the concept of “this” keyword in JavaScript.
7. What is the Document Object Model (DOM)?
8. What is the difference between localStorage and sessionStorage in HTML5?
9. What are the advantages and disadvantages of using AJAX in web development?
10. What are some techniques for optimizing website accessibility?
Advanced:
1. What are Web Components, and how do they differ from traditional HTML elements?
2. Explain the concept of Virtual DOM and its advantages in frameworks like React.
3. What are some strategies for improving website security?
4. What are some features of ES6 (ECMAScript 2015), and how do they improve JavaScript development?
5. What is the difference between client-side rendering and server-side rendering?
6. Explain the concept of state management in front-end frameworks like React or Vue.js.
7. What are some techniques for optimizing web applications for mobile devices?
8. How do you handle cross-browser compatibility issues in web development?
9. Explain the concept of progressive web apps (PWAs) and their benefits.
10. What are some performance optimization techniques specific to single-page applications (SPAs)?
Basic Front end developer interview questions :
1. HTML (Hypertext Markup Language):
– HTML is the standard markup language for creating web pages and web applications. It defines the structure and layout of a web document by using a variety of tags and attributes.
<!DOCTYPE html>
<html>
<head>
<title>My First HTML Page</title>
</head>
<body>
<h1>Hello, World!</h1>
<p>This is a paragraph.</p>
</body>
</html>
2. CSS (Cascading Style Sheets):
– CSS is a style sheet language that is used to describe the presentation of a document written in HTML. It controls the layout, colors, fonts, and other visual aspects of a web page.
/* CSS Stylesheet */
body {
background-color: #f0f0f0;
font-family: Arial, sans-serif;
}
h1 {
color: blue;
}
3. Inline, Block, and Inline-block elements in CSS:
– Inline elements: These elements do not start on a new line and only take up as much width as necessary. Examples include `<span>` and `<a>`.
– Block elements: These elements start on a new line and take up the full width available. Examples include `<div>`, `<p>`, and `<h1>`.
– Inline-block elements: These elements are similar to inline elements as they do not start on a new line, but they can have width and height properties like block elements. Examples include `<img>` and `<button>`.
<!-- HTML Example -->
<span style="color: red;">Inline Element</span>
<div style="background-color: blue;">Block Element</div>
<button style="display: inline-block;">Inline-block Element</button>
4. Box Model in CSS:
– The box model in CSS describes the design and layout of elements on a web page. It consists of content, padding, border, and margin.
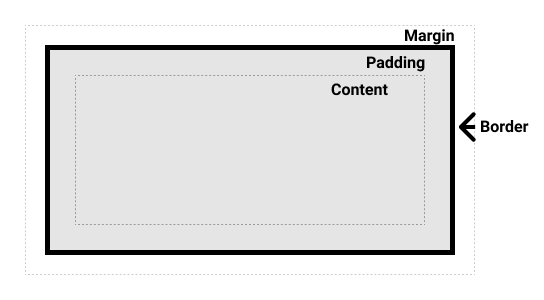
/* CSS Example */
.box {
width: 200px;
height: 100px;
padding: 20px;
border: 2px solid black;
margin: 10px;
}
5. Purpose of JavaScript in Web Development:
– JavaScript is a programming language that enables interactive and dynamic features on web pages. It can be used to manipulate HTML and CSS, handle events, create animations, and communicate with servers.
// JavaScript Example
document.getElementById("myButton").addEventListener("click", function() {
document.getElementById("demo").innerHTML = "Hello, World!";
});
These examples provide a basic understanding of HTML, CSS, and JavaScript and their roles in web development.
Sure, here are detailed explanations along with code examples for each question:
6. Data types in JavaScript:
JavaScript has several data types including:
– Primitive Data Types: Number, String, Boolean, Undefined, Null
– Complex Data Types: Object, Function
// Primitive Data Types
let num = 10; // Number
let str = "Hello"; // String
let bool = true; // Boolean
let undef; // Undefined
let nul = null; // Null
// Complex Data Types
let obj = { key: "value" }; // Object
function func() { console.log("Function"); } // Function
7. Difference between == and === in JavaScript:
– `==` is the equality operator that performs type coercion, meaning it will attempt to convert operands to the same type before making the comparison.
– `===` is the strict equality operator that checks both the value and the type without performing type coercion.
let num = 10;
let strNum = "10";
console.log(num == strNum); // true, because '10' gets coerced to 10
console.log(num === strNum); // false, because they are of different types
8. CSS preprocessor:
A CSS preprocessor is a scripting language that extends CSS and compiles it into regular CSS. It adds features like variables, nesting, and mixins, which can make CSS code more maintainable and efficient.
/* SCSS syntax example */
$primary-color: #007bff;
.button {
background-color: $primary-color;
&:hover {
background-color: darken($primary-color, 10%);
}
}
9. Responsive web design:
Responsive web design is an approach to web design that makes web pages render well on a variety of devices and window or screen sizes. It involves using flexible grids and layouts, images, and CSS media queries.
10. Purpose of media queries in CSS:
Media queries in CSS allow you to apply different styles for different devices and conditions, such as screen size, device orientation, and resolution. They are commonly used in responsive web design to adjust layout and styling based on the characteristics of the device viewing the page.
/* Example of a media query */
@media (max-width: 600px) {
body {
font-size: 14px;
}
}
Intermediate Front end developer interview questions :
1. Differences between GET and POST requests:
– GET: Used to request data from a specified resource. Data is sent in the URL, visible to everyone. Limited amount of data can be sent.
<form action="/submit" method="GET">
<input type="text" name="username">
<input type="submit" value="Submit">
</form>
– POST: Used to submit data to be processed to a specified resource. Data is sent in the request body, not visible in the URL. Can send large amounts of data.
<form action="/submit" method="POST">
<input type="text" name="username">
<input type="submit" value="Submit">
</form>
2. Closures in JavaScript:
– A closure is a combination of a function bundled together with references to its surrounding state (lexical environment). It gives you access to an outer function’s scope from an inner function.
function outerFunction() {
let outerVariable = 'I am outer';
function innerFunction() {
console.log(outerVariable); // Accessing outerVariable from outer function
}
return innerFunction;
}
let closureFunc = outerFunction();
closureFunc(); // Output: I am outer
3. Event delegation in JavaScript:
– Event delegation is a technique where a single event listener is attached to a parent element to manage events on its children. This is useful when dynamically adding or removing child elements.
<ul id="parent-list">
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
<script>
document.getElementById('parent-list').addEventListener('click', function(e) {
if (e.target.tagName === 'LI') {
console.log('Clicked on: ', e.target.textContent);
}
});
</script>
4. Optimizing website performance:
– Minimize HTTP requests
– Minify and combine files
– Use asynchronous loading for CSS and JavaScript
– Optimize images
– Enable compression (e.g., Gzip)
– Use a content delivery network (CDN)
– Reduce server response time
– Use caching
– Implement lazy loading
– Optimize CSS and JavaScript
– Monitor and analyze performance regularly
5. Common CSS frameworks and libraries:
– Bootstrap: Provides pre-styled components and grid system for responsive layouts. Useful for quickly prototyping or building modern websites.
– Foundation: Similar to Bootstrap, offering a grid system and UI components. Known for its flexibility and customization options.
– Bulma: A lightweight CSS framework with a modern look and feel. Focuses on simplicity and responsiveness.
– Tailwind CSS: Utility-first CSS framework, where you build designs by composing utility classes. Offers great flexibility and customization.
– Materialize CSS: Based on Google’s Material Design principles, offering ready-to-use components and a responsive grid system.
– Semantic UI: A development framework that uses human-friendly HTML to build responsive layouts. It emphasizes natural language principles.
6. State Management in Front-end Frameworks:
State management in front-end frameworks like React or Vue.js refers to the management and manipulation of the application’s state, which includes data that determines the behavior and appearance of the user interface. These frameworks typically follow a unidirectional data flow architecture, where data flows in a single direction, from parent components to child components.
In React, state management is often handled using the built-in `useState` hook for functional components or the `setState` method for class components. Additionally, React offers solutions like Redux or Context API for managing complex state across multiple components.
In Vue.js, state management can be achieved using the `data` option within Vue instances for local component state, or Vuex for managing global state across the application.
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
};
export default Counter;
7. Optimizing Web Applications for Mobile Devices:
Optimizing web applications for mobile devices involves various techniques to ensure a smooth and responsive user experience on smaller screens and potentially slower network connections. Some techniques include:
– Responsive Design: Using CSS media queries to adjust the layout and styling of the application based on the screen size and orientation.
– Viewport Meta Tag: Setting the viewport meta tag to control the layout viewport width and scale on mobile devices.
– Optimizing Images: Using responsive images and techniques like lazy loading to reduce page load times and bandwidth usage.
– Minifying and Compressing Resources: Minifying CSS, JavaScript, and HTML files, as well as compressing images and other assets to reduce file sizes and improve loading times.
– Reducing HTTP Requests: Combining multiple CSS and JavaScript files, using CSS sprites, and minimizing the number of external resources to reduce the number of HTTP requests.
– Touch-friendly UI: Designing touch-friendly interfaces with larger buttons and clickable elements to accommodate mobile users.
– Progressive Web App (PWA) Features: Implementing PWA features like service workers for offline support and app-like experiences on mobile devices.
8. Handling Cross-Browser Compatibility Issues:
Cross-browser compatibility issues arise when a website or web application behaves differently across different web browsers due to variations in browser rendering engines and standards support. To handle these issues, developers can employ the following techniques:
– Browser Testing: Regularly testing websites and applications across different browsers and versions to identify compatibility issues.
– Feature Detection: Using feature detection libraries like Modernizr to detect browser capabilities and apply appropriate fallbacks or polyfills.
– Prefixing CSS Properties: Using vendor prefixes (-webkit-, -moz-, -ms-, etc.) for CSS properties to ensure compatibility with different browsers.
– Polyfills: Using polyfills to add support for newer JavaScript features in older browsers that may not natively support them.
– Using CSS Resets or Normalizers: Employing CSS resets or normalizers to ensure consistent rendering across different browsers by resetting or normalizing default styles.
– Progressive Enhancement: Building websites and applications with a progressive enhancement approach, starting with a basic functional version and enhancing it with advanced features for modern browsers.
9. Progressive Web Apps (PWAs) and Their Benefits:
Progressive Web Apps (PWAs) are web applications that leverage modern web technologies to provide a native app-like experience to users, including offline functionality, push notifications, and device hardware access. Some benefits of PWAs include:
– Offline Support: PWAs can work offline or with a poor internet connection using service workers to cache assets and data.
– Responsive Design: PWAs are built with responsive design principles, ensuring they work seamlessly across different devices and screen sizes.
– Improved Performance: PWAs are designed to be fast and efficient, providing a smooth user experience with quick loading times and smooth animations.
– Engagement: PWAs can engage users with features like push notifications, allowing businesses to re-engage users and increase user retention.
– Installation: PWAs can be installed on a user’s device, appearing on the home screen without requiring a visit to an app store.
– Cost-Effectiveness: Developing a single PWA that works across multiple platforms can be more cost-effective than developing separate native apps for each platform.
10. Performance Optimization Techniques for Single-Page Applications (SPAs):
Single-Page Applications (SPAs) are web applications that dynamically update the current page rather than loading entire new pages from the server. To optimize the performance of SPAs, developers can employ various techniques such as:
– Code Splitting: Splitting the application code into smaller chunks and loading them asynchronously to reduce initial load times.
– Lazy Loading: Loading non-essential resources, such as images or components, only when they are needed, rather than loading everything upfront.
– Client-Side Routing: Using client-side routing libraries to handle navigation within the SPA, avoiding full-page reloads and improving user experience.
– Optimizing Bundle Size: Minifying and compressing JavaScript bundles, as well as optimizing images and other assets to reduce file sizes.
– Caching and Prefetching: Utilizing browser caching and prefetching strategies to cache assets and data, reducing server round-trips and improving performance.
– Virtual DOM: Leveraging virtual DOM implementations, like those in React or Vue.js, to efficiently update the UI and minimize DOM manipulation overhead.
– Performance Monitoring and Tuning: Regularly monitoring application performance using tools like Chrome DevTools or Lighthouse, and optimizing performance based on the findings.
// Example of lazy loading in React using React.lazy and Suspense
import React, { Suspense } from 'react';
const LazyComponent = React.lazy(() => import('./LazyComponent'));
const App = () => {
return (
<div>
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
</div>
);
};
export default App;
Advance Front end developer interview questions :
1. Web Components vs. Traditional HTML Elements:
Web Components are a set of web platform APIs that allow you to create new custom, reusable, encapsulated HTML tags to use in web pages and web apps. They consist of four main technologies: Custom Elements, Shadow DOM, HTML Templates, and HTML Imports. Unlike traditional HTML elements, Web Components provide a way to encapsulate functionality and styling, making them more modular and reusable across different projects.
<template id="my-template">
<style>
/* Styles for the component */
</style>
<div>
<!-- Markup for the component -->
</div>
</template>
<script>
class MyComponent extends HTMLElement {
constructor() {
super();
const template = document.getElementById('my-template').content;
this.attachShadow({ mode: 'open' }).appendChild(template.cloneNode(true));
}
}
customElements.define('my-component', MyComponent);
</script>
2. Virtual DOM in React:
Virtual DOM is a concept used in frameworks like React to improve performance and efficiency when updating the user interface. It’s a lightweight copy of the actual DOM tree maintained by the framework. When there are changes in the application’s state, React updates the Virtual DOM instead of directly manipulating the real DOM. Then, it calculates the most efficient way to update the real DOM and applies those changes in a batch. This approach reduces unnecessary reflows and repaints, leading to faster UI updates.
// Example React component demonstrating Virtual DOM
class MyComponent extends React.Component {
render() {
return <div>{this.props.message}</div>;
}
}
3. Strategies for Website Security:
Improving website security involves various strategies, including:
– Implementing HTTPS to encrypt data transmitted between the server and the client.
– Using strong authentication mechanisms like multi-factor authentication (MFA).
– Validating and sanitizing user input to prevent injection attacks like SQL injection and Cross-Site Scripting (XSS).
– Keeping software dependencies and frameworks up-to-date to patch known vulnerabilities.
– Employing proper access controls to limit user privileges and prevent unauthorized access to sensitive data.
– Regularly monitoring and logging website activities to detect and respond to security incidents promptly.
4. Features of ES6 and JavaScript Development:
ES6 introduced several features to JavaScript, such as arrow functions, template literals, let and const for variable declaration, classes, destructuring assignment, spread/rest operators, and promises. These features improve JavaScript development by making the code more concise, readable, and maintainable. They also introduce modern programming paradigms and patterns, enabling developers to write more efficient and expressive code.
// Example of ES6 features
const name = 'John';
console.log(`Hello, ${name}!`); // Template literals
const add = (a, b) => a + b; // Arrow function
console.log(add(2, 3)); // Output: 5
5. Client-side Rendering vs. Server-side Rendering:
Client-side rendering involves rendering web pages on the client side (in the browser) using JavaScript frameworks like React, Angular, or Vue.js. In this approach, the server sends raw data (usually in JSON format), and the client-side JavaScript code renders the UI dynamically based on that data. This leads to faster initial page loads but may result in slower subsequent interactions as the client needs to download and execute JavaScript code.
Server-side rendering, on the other hand, involves rendering web pages on the server side before sending them to the client. The server generates the complete HTML content for each page and sends it to the client’s browser. This approach provides faster perceived loading times, better SEO (Search Engine Optimization), and improved performance on devices with limited resources. However, it may require more server resources and can be less interactive compared to client-side rendering.
// Example of client-side rendering with React
function App() {
return <h1>Hello, World!</h1>;
}
ReactDOM.render(<App />, document.getElementById('root'));
// Example of server-side rendering with React
const express = require('express');
const React = require('react');
const ReactDOMServer = require('react-dom/server');
const App = require('./App');
const app = express();
app.get('/', (req, res) => {
const html = ReactDOMServer.renderToString(<App />);
res.send(html);
});
app.listen(3000, () => {
console.log('Server is listening on port 3000');
});
6. State Management in Front-end Frameworks:
State management in front-end frameworks like React or Vue.js refers to the management of the application’s data/state in a centralized location that can be accessed and modified by different components. This ensures that the application remains consistent and predictable as it grows in complexity.
Example (React using useState hook):
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
7. Optimizing Web Applications for Mobile Devices:
Some techniques for optimizing web applications for mobile devices include:
– Responsive design using CSS media queries
– Optimizing images and media for mobile screens
– Minimizing HTTP requests
– Implementing touch events instead of mouse events
– Utilizing browser caching
8. Handling Cross-Browser Compatibility Issues:
To handle cross-browser compatibility issues, developers can:
– Use modern web standards and best practices
– Test the application on multiple browsers and devices
– Utilize polyfills to provide functionality in older browsers
– Use feature detection libraries like Modernizr
– Regularly update and maintain the codebase to adapt to new browser versions
9. Progressive Web Apps (PWAs) and Their Benefits:
Progressive Web Apps (PWAs) are web applications that provide a native app-like experience to users while being built using web technologies. They offer benefits such as:
– Offline capabilities through service workers
– Fast loading times and smooth performance
– Push notifications
– Responsive design for various devices
– Discoverability through search engines
10. Performance Optimization Techniques for Single-Page Applications (SPAs):
Some performance optimization techniques specific to SPAs include:
– Code splitting to load only necessary code chunks
– Lazy loading of components and resources
– Minification and compression of JavaScript and CSS files
– Implementing client-side caching strategies
– Using virtual scrolling and infinite loading for large lists or data sets
Also Read: Top 30 JavaScript Interview Questions And Answers For 2024
Front end developer interview questions 2024Front end developer interview questions 2024Front end developer interview questions 2024Front end developer interview questions 2024Front end developer interview questions 2024Front end developer interview questions 2024Front end developer interview questions 2024Front end developer interview questions 2024Front end developer interview questions 2024Front end developer interview questions 2024Front end developer interview questions 2024Front end developer interview questions 2024Front end developer interview questions 2024